1.
Construct a binary tree using the following sequences:
Inorder: N, M, P, O, Q
Postorder: N, P, Q, O, M
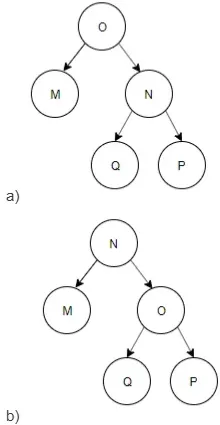
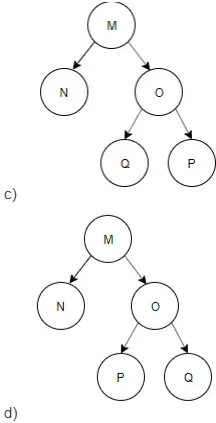
2.
What does a digital twin represent in IoT?
3.
Which directive is used to define macros in Embedded C?
4.
Which of the following is a correct way to implement a thread-safe singleton in C++11?
5.
What will be the output of the following code snippet?
var a = true + true + true * 3;
print(a)
6.
Which data structure is used for implementing LRU (Least Recently Used) cache?
7.
In Node.js, which of the following is an in-built event emitter?
8.
Which of the following is true about std::shared_ptr?
9.
What does LPWAN stand for in IoT networks?
10.
Which of the following are not server-side Javascript objects?
11.
What is the default port number used by the MQTT protocol?
12.
What is the Purpose of the Next Parameter in Express.js Middleware Functions?
13.
Which statement is incorrect regarding binary trees?
14.
Which of the following is a key feature of CoAP (Constrained Application Protocol)?
15.
What is normalization in the context of databases?
16.
The prefix form of A-B/(C*D^E) is?
17.
What is the worst case time complexity of a sequence of n queue operations using the Multiqueue operation?
Multiqueue(Q)
{
m=k;
while(Q is not empty) and (m > 0)
{
DEQUEUE(Q)
m=m-1
}
}
18.
What does the emplace_back method do in a std::vector?
19.
Which sorting algorithm does std::sort use by default?
20.
In an embedded system, what does the volatile keyword indicate?
21.
Which IoT platform is provided by Amazon?
22.
What is used to handle code-splitting?
23.
How do you Apply Middleware to Specific Routes in Express.js?
24.
In which case is std::move most useful?
25.
What is the function of a message broker in IoT systems?
26.
What is the output of the following code snippet?
#include
#include
int main() {
std::vector v = {1, 2, 3, 4};
for (int &x : v) {
x *= 2;
}
for (int x : v) {
std::cout << x << " ";
}
return 0;
}
27.
Which of the following concepts make extensive use of arrays?
28.
What is the functionality of this code?
public int function(int data)
{
Node temp = head;
int var = 0;
while(temp != null)
{
if(temp.getData() == data)
{
return var;
}
var = var+1;
temp = temp.getNext();
}
return Integer.MIN_VALUE;
29.
What does the noexcept specifier indicate in C++?
30.
What is the primary purpose of the std::enable_if template in C++?
31.
In a binary search tree, what is the time complexity of finding the maximum or minimum element?
32.
What is the primary role of a gateway in an IoT ecosystem?
33.
In a balanced binary tree, the height difference between left and right subtrees for every node is:
34.
In IoT, what is the purpose of the publish-subscribe model?
35.
Which of the following is an advantage of using interrupts in Embedded C?
36.
What is the correct order of the phases in an embedded C program development cycle?
37.
What happens when a pointer to a NULL address is dereferenced in an embedded system?
38.
Which MongoDB command removes all documents from a collection without dropping it?
39.
Which series will be printed by the following pseudocode?
Integer a, b, c
Set b = 4, c = 5
for(each a from 2 to 4)
print c
b = b - 1
c = c + b
end for
40.
What is the purpose of ISR in an embedded system?
41.
Which of the following can cause undefined behavior in C++?
42.
Which of the following protocols is commonly used for inter-device communication in embedded systems?
43.
What is the time complexity of finding an element in std::unordered_map?
44.
Which of the following is the correct syntax for a lambda function in C++?
45.
Which of the following is the best data structure for implementing a priority queue?
46.
Which of the following sorting algorithms has the best worst-case time complexity?
47.
What will be the output of the following code snippet?
function(){
setTimeout(()=> console.log(1),2000);
console.log(2);
setTimeout(()=> console.log(3),0);
console.log(4);
})();
48.
Which programming language is most commonly used for IoT firmware development?
49.
What is the size of a char in most embedded systems?
50.
When is useReducer used over useState in React component?
51.
Which of the following terms commonly described react applications?
52.
What is the time complexity of inserting an element into a sorted linked list?
53.
Which protocol provides device-to-device communication in IoT networks?
54.
What will be the output of the following code snippet? std::string s = "Hello"; std::reverse(s.begin(), s.end()); std::cout
55.
Which data structure is used for implementing recursion?
56.
What command is used to insert a document into a collection in MongoDB?
57.
Which of the following is NOT a valid storage class in Embedded C?
58.
What is the maximum number of symbols that will appear on the stack AT ONE TIME during the conversion of this infix expression to postfix: 4 + 3*(6*3-12)?
59.
What is the role of a device shadow in IoT?
60.
What is the significance of edge computing in IoT?
61.
Which of the following is used to access a function fetch()Â from h1 element in JSX?
62.
Which of the following statements is true about MongoDB's schema design?
63.
What is the difference between process.nextTick() and setImmediate()?
64.
What is the maximum number of nodes in a binary tree of height h?
65.
In a typical embedded system, what is the primary purpose of a watchdog timer?
67.
Choose the component which should be overridden to stop the component from updating?
68.
What is the advantage of std::function over raw function pointers?
69.
Which encryption method is commonly used to secure IoT device communication?
70.
How does std::unique_ptr differ from std::shared_ptr?
71.
What is the Event Loop in Node.js?
72.
What is the functionality of this code?
public void function(Node node)
{
if(size == 0)
head = node;
else
{
Node temp,cur;
for(cur = head; (temp = cur.getNext())!=null; cur = temp);
cur.setNext(node);
}
size++;
}
73.
Which protocol is most commonly used for lightweight communication in IoT?
74.
What is the best-case time complexity of Quick Sort?
75.
What is the primary advantage of a doubly linked list over a singly linked list?
76.
What does the term "collision" mean in the context of hash tables?
77.
What will be the output of the following code snippet?
var a = "hello";
var sum = 0;
for(var i = 0; i < a.length; i++) {
sum += (a[i] - 'a'); }
print(sum);
78.
Which of the following traversal techniques visits nodes level by level in a tree?
79.
Which IoT board is best suited for low-power, battery-operated applications?
80.
How do we send the message from one socket to another socket in Node.js?